Hey Chris, to handle a checkbox and check its status using Selenium Webdriver, follow these steps:
1. Launch the Eclipse IDE and right click on the src folder and then click on the New > class. Enter the class name as Checkbox_test:
2. Now, invoke the Google Chrome browser by setting the system property to the path of your chromedriver.exe file:
System.setProperty("webdriver.chrome.driver","C:\\\\work\\\\chromedriver.exe);
WebDriver driver = new ChromeDriver();
3. Next, navigate to the SpiceJet website:
driver.navigate().to("https://www.example.com/");
4. Then try to locate the 'Senior Citizen' checkbox by inspecting its HTML code:
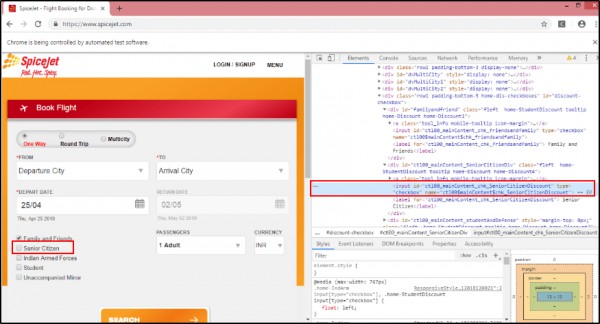
5. Write the code that will locate 'Senior Citizen' checkbox and check whether its checked or not by using these line of code:
System.out.println(driver.findElement(By.cssSelector("input[id*='SeniorCitizenDiscount']")).isSelected());
driver.findElement(By.cssSelector("input[id*='SeniorCitizenDiscount']")).click();
System.out.println(driver.findElement(By.cssSelector("input[id*='SeniorCitizenDiscount']")).isSelected());
6. Finally, click on Run button to execute the automation. You would see this result in your Console:
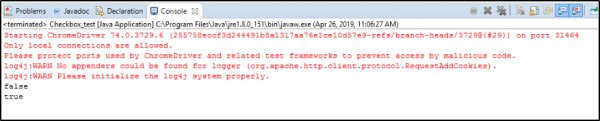