Pandas provide data analysts a way to delete and filter data frames using a data frames.drop() method. We can use this method to drop such rows that do not satisfy the given conditions.
Example 1: Delete rows based on condition on a column.
# import pandas library
import pandas as pd
# dictionary with list object in values
details = {
'Name' : ['Ankit', 'Aishwarya', 'Shaurya',
'Shivangi', 'Priya', 'Swapnil'],
'Age' : [23, 21, 22, 21, 24, 25],
'University' : ['BHU', 'JNU', 'DU', 'BHU',
'Geu', 'Geu'],
}
# creating a Dataframe object
df = pd.DataFrame(details, columns = ['Name', 'Age',
'University'],
index = ['a', 'b', 'c', 'd', 'e', 'f'])
# get names of indexes for which
# column Age has value 21
index_names = df[ df['Age'] == 21 ].index
# drop these row indexes
# from dataFrame
df.drop(index_names, inplace = True)
df
Output :
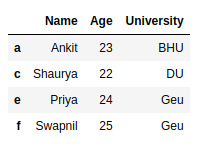